As of 2023, approximately 90% of organizations implementing DevOps practices have adopted infrastructure as code (IaC) to some extent (1). This adoption spans various industries, including technology, finance, healthcare, and retail (2). Companies adopt IaC to deploy infrastructure faster, with fewer errors, for a lower cost than they do without it.
However, there are challenges when adopting IaC:
- Skill Gaps: 60% of organizations cite a lack of skilled professionals as a significant barrier to fully implementing IaC practices (3)
- Security Concerns: Ensuring security and compliance through IaC remains a top concern for 50% of organizations, emphasizing the importance of integrating security best practices into IaC (4).
While the benefits are obvious, surmounting these challenges isn’t so straightforward. Many companies try to build their own infrastructure as code templating. In this overview we’ll run through some of the options available today, and introduce Resourcely: a tool built for infrastructure as code templating.
Infrastructure as code templating
Given infrastructure as code is….code, it is relatively easy to parameterize that code and allow users to make inputs into it without having to write the full code themselves. This has emerged as the most effective way to help developers deploy infrastructure in the new world of DevOps (where they are expected to do just that).
Generally, Platform, DevOps, and SRE teams create infrastructure as code modules or templates by defining reusable components that encapsulate specific infrastructure patterns. These often feature variables or parameters that can take input from end-users in order to customize the resulting IaC.
In practice, templating gets particularly sticky around:
- Learning Curve: While most IaC is declarative and designed to be human-readable, mastering the syntax can be challenging for newcomers.
- Limited Procedural Logic: Declarative languages advanced procedural programming constructs, potentially limiting complex automation scenarios.
- Cloud Infrastructure Complexity: IaC itself doesn’t solve the inherent problem of cloud infrastructure complexity. There are thousands of possible cloud resources, each with 10 - 100 different configuration possibilities. This heterogeneity is one of the primary causes of the frustration with tools like Terraform and CloudFormation.
Terraform
Terraform is the most widely adopted of IaC languages, with a modular architecture with support for nearly every major cloud provider that has made it incredibly popular.
Example: Simple Terraform Module that provisions an S3 bucket
Usage:
Illustrating Terraform Module Complexities
Let's examine a more complex Terraform module that can be difficult for end-user developers to understand and use effectively.
Example: AWS VPC with Multiple Resources
Usage:
Using this module would be difficult, even for the most experienced of developers. It…
- requires the user to input multiple variables, including lists for subnets and availability zones. Managing and understanding these variables can be overwhelming for developers unfamiliar with networking concepts.
- assumes a specific structure and number of public and private subnets corresponding to the availability zones. Mismatched lengths between
public_subnets
,private_subnets
, andavailability_zones
can lead to errors that are not immediately clear. - doesn’t have comprehensive or embedded documentation, meaning end-users may struggle to understand the purpose of each variable and how to configure it properly.
- does not expose all possible configurations, limiting the end-user’s ability to tailor the infrastructure to their specific needs. This can force users to modify the module directly, which defeats the purpose of reusability and can introduce maintenance challenges.
- has a complex code structure, making it harder for end-users to quickly grasp its functionality. Looking at this example, your eyes will almost surely become bleary.
Imagine an end-user developer attempts to use our advanced_vpc
module but provides mismatched lists for public_subnets
, private_subnets
, and availability_zones
:
You can imagine this scenario happening, because it is a very possible one! If it were to happen, the terraform plan
would fail with an error message like:
Terraform modules don’t suffer from the problem of poor design. They are doing what they are designed for: helping cloud infrastructure experts move faster. Let’s cover some other tooling that is templated for configuring infrastructure:
AWS CloudFormation
Another popular IaC framework specifically for AWS is CloudFormation. CloudFormation templates use declarative JSON or YAML files (similar to Terraform).
Here’s an example of a CloudFormation template written in YAML. It takes parameters from a developer and creates an EC2 instance. You can see some of the customization available in the template parameters (allowed values, description, type, default, etc.):
Interacting with this template would require using the AWS CLI or CDK. Here’s an example of how a developer would use it using the CLI:
Similar to Terraform modules, CloudFormation templates are not inherently modular, and can become very verbose. When linking together templates, deployment order becomes important and dependency hell can cause even the most experienced AWS developers to give up in frustration.
Other infrastructure as code templating
Ansible is an agentless automation tool that uses YAML-based playbooks to define and manage configurations, deployments, and orchestration tasks. Given it is YAML-based, templating support for Ansible is with Jinja2.
Pulumi allows developers to define infrastructure using general-purpose programming languages like Python, TypeScript, and Go. While Pulumi provides templates on their website, those are general-purpose patterns. Given the variable language flexibility, developers must be familiar with that language in order to use it.
Chef uses a Ruby-based DSL (Domain-Specific Language) to define infrastructure configurations. Templating for Ruby-based frameworks is accomplished using Embedded Ruby (ERB), which can be prohibitive if your developer base isn’t fluent in Ruby.
SaltStack is an event-driven automation tool that uses YAML-based state files to define desired infrastructure states. Similar to Ansible, it is templated using Jinja2 without any specific quality-of-life features.
Infrastructure as code templating drawbacks
The primary issue with the infrastructure as code templating options available, is that they don’t go far enough. At their most basic (Jinja2), they allow for collection and insertion of inputs. At their most advanced (Terraform modules), they allow for defaults, descriptions, enums, types, etc.
All of these infrastructure as code templating options do not:
- embed any automated typing
- automatically detect linking between resources
- provide a UI
- automatically provide defaults for enums
Resourcely
Resourcely is a cloud configuration platform built to solve the cloud complexity problem. It both allows for IaC templating and maintains a knowledge graph of cloud resource metadata that makes creating and using IaC templates faster, simpler, and easier.
Resourcely Templating
Resourcely supports templating of Infrastructure as Code with a Liquid-style syntax.
Features that you would expect from templates (defaults, descriptions, etc) are included, while advanced quality-of-life concepts that utilize a proprietary knowledge graph help overcome cloud complexity. This includes automatic linking of resources, enum detection, parameterization, context-dependent options, and more are supported out of the box.
You can read more about building templates with Resourcely here.
Foundry (IaC Templating IDE)
Resourcely templates are created using Foundry: an integrated IDE for building, previewing, and testing templates. Platform teams report that with Resourcely, they can reduce their template creation time by 90% while helping developers ship infrastructure 80% faster.
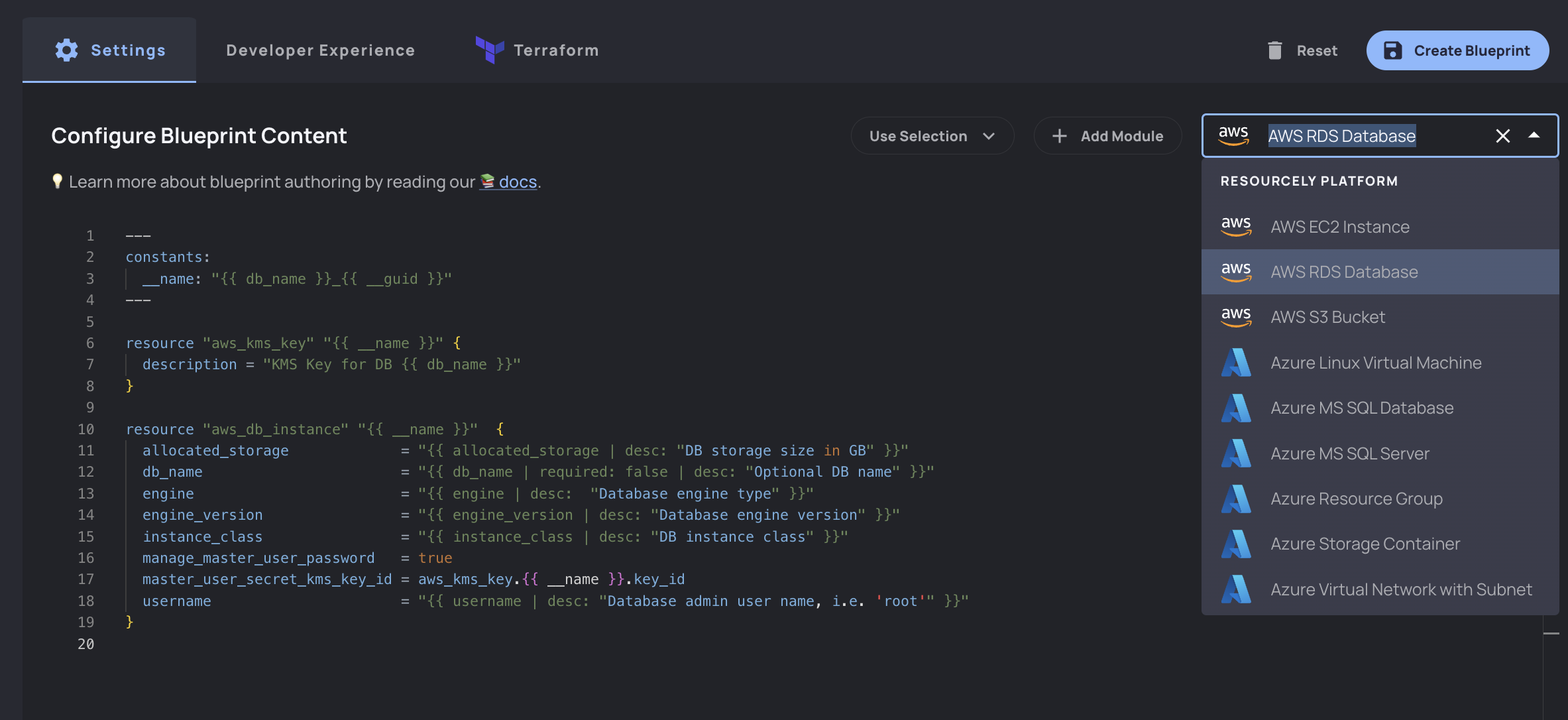
Template UI
Every template (Blueprint, in Resourcely parlance) becomes an automatically hosted UI that developers can interact with to generate Infrastructure as Code. This UI includes a service catalog-style shopping cart where developers can build their own custom infrastructure stack from linkable, modular components.
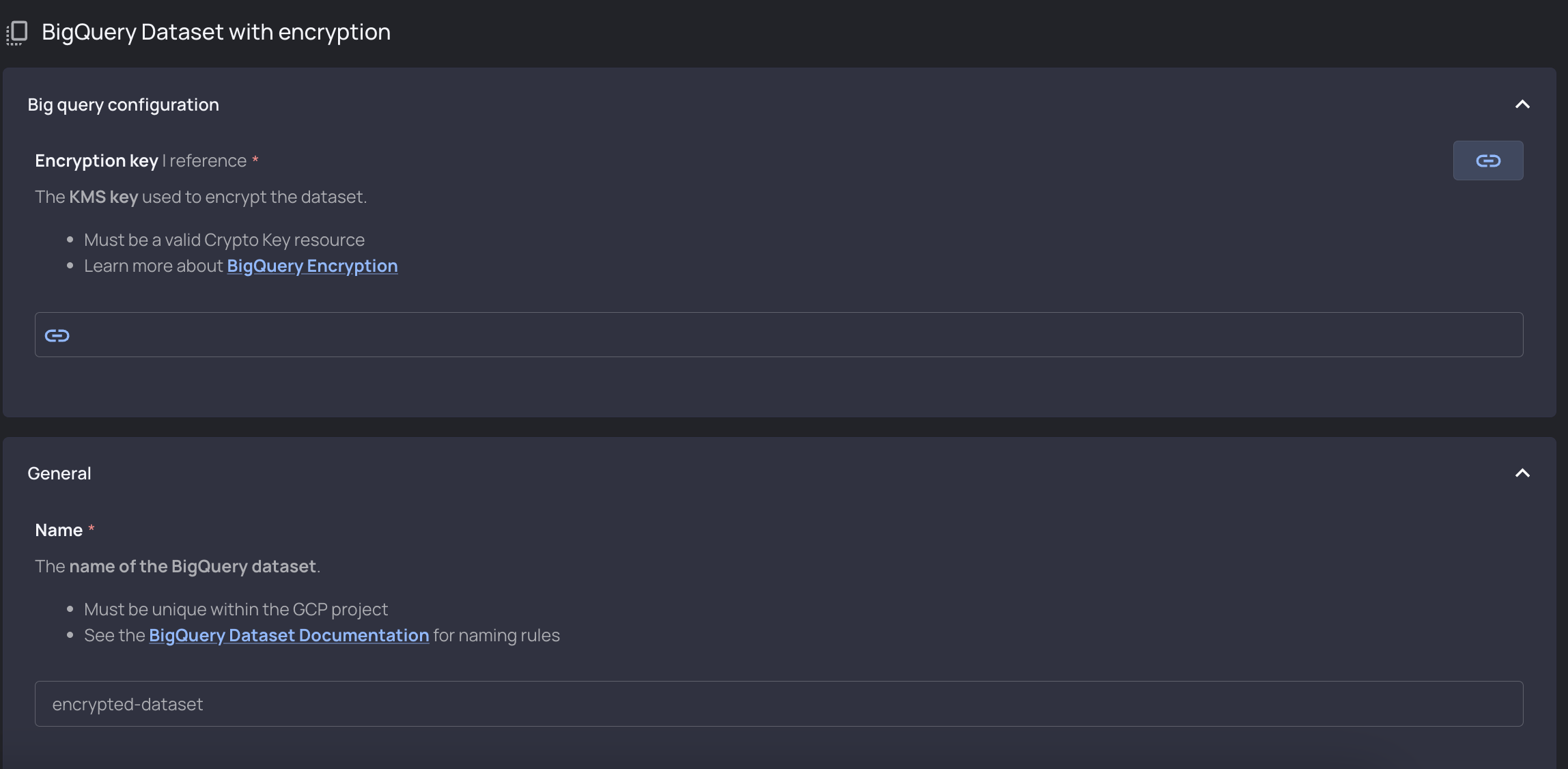
Infrastructure Policy-as-Code
Resourcely also embeds infrastructure policy-as-code, allowing platform teams to control the infrastructure configuration that developers create with Resourcely templates. These policies are exposed to developers within the Resourcely UI, so that they don’t find out about policy violations after CI runs.
Conclusion
When evaluating an IaC templating tool, it is important to think about the developer experience of interacting with that template or module. Existing templating tools require a significant amount of effort by platform teams to build user-friendly modules.
Resourcely offers a next-generation infrastructure templating framework, with cloud resource parameter information embedded along with an automatically hosted UI. This is a unique alternative to Terraform modules or CloudFormation templates, which rely on platform teams to codify cloud complexity on their own.
References
- (1). DevOps Survey 2023. https://puppet.com/resources/report/devops-survey-2023/
- (2). State of the Cloud Report 2023. https://info.flexera.com/CM-REPORT-State-of-the-Cloud
- (3). State of DevOps Report 2023. https://cloud.google.com/devops/state-of-devops
- (4). State of the Cloud Report 2024. https://info.flexera.com/CM-REPORT-State-of-the-Cloud